What is Einstein OCR?
We all know that OCR is an Optical Character Recognition which can detect and extract text from images. But, what about Einstein OCR?
Einstein OCR provides models that can detect text in an image such as Business cards, Images that contain unformatted data and images that contain data in tables.
In this blog post, we will focus on the Business Card Model. When choosing business card model, Einstein OCR can detect the entity type text such as Person, Organization, Email, Phone, Address, etc...
Sign Up
To get Started with Einstein Vision, you first need to register for an Account (If you don't already have one).
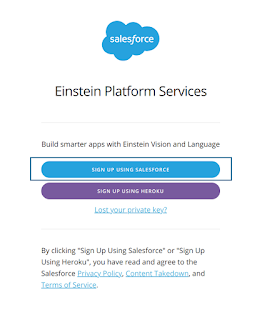 |
Sign Up
|
It is quite straight forward after that. If all goes well, you should see a screen with your Einstein Private Key like below. Download the key and keep it safe for later.
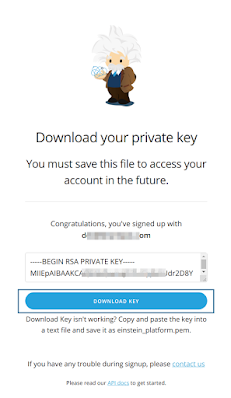 |
Private Key
|
Setup
Deploy Salesforce code
Once you have an Einstein Account, we can start the setup. For the next step, you will require some basic developer skills.
Connect the project to your Organization using SFDX: Authorize an Org option and then deploy all the elements inside the project structure.
Configure Permission for Salesforce App
When everything has been deployed, you can assign the App Einstein OCR to the desired Profile.
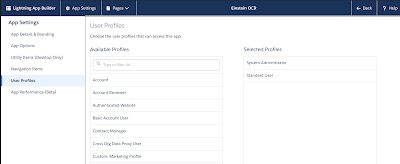 |
Profile Assignment
|
Setup Einstein Vision
Configuring Einstein Vision is pretty straight forward, just switch to the Einstein OCR app on your Salesforce and open the Einstein Vision Setup tab
Enter the email address you used to Sign Up (make sure it is the email and not the Salesforce Username; you should find it in the welcome Einstein Mail).
Then use the Upload Files button to upload the private key (einstein_platform.pem file that you downloaded previously)
When saving the setting, you should have a green tick icon on the right hand side of the component to validate that the Account is valid.
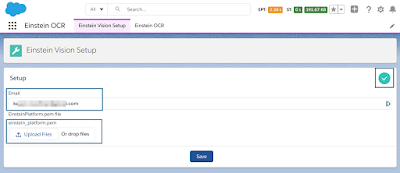 |
Setup Einstein Vision |
OCR in Action
To see the OCR in action, simply click on the Einstein OCR tab next to the Einstein Vision Setup tab.
Insert a Business Card Image Url and click on Scan.
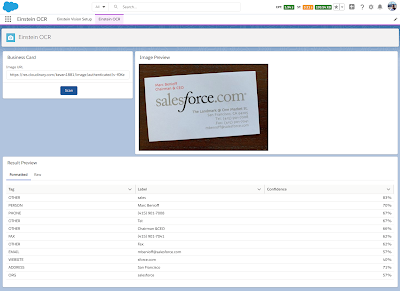 |
Demo
|
The page comprises of 3 simple component:
- Component to input the Image Url
- Another one for Preview the Business Card
- lastly, result previewer
From the above example, you can see Mark Benioff business card. It has already categorised the different elements found on the Business Card.
Wrap-Up
If you want to dive into more details on how the API call is done and detection result is processed, you can just take a look at the class EinsteinVisionUtils and Prediction class in the source code.